Ethereum: Decoding the leverage_bracket
function using the Binance API
Introduction
When using the Binance API, you can get the response as a list of strings. In this article, we will look at how to extract and use the initialLeverage' variable from this response.
Problem: extracting the variable 'initialLeverage'
Assume that your API response contains the following data:
[
{
"leverage_bracket": [
{ "side": "BUY", "amount": 100 },
{ "side": "SELL", "amount": 200 }
]
}
]
In this case, initialLeverageis the key in the first dictionary in the list.
Extracting and using 'initialLeverage'
You can modify your code to parse the response string to extract and use theinitialLeveragevalue. Let's assume that the API response contains only one element in the array, so we'll access it using index 0.
import json
def long():
"""
A function to simulate a long position on Ethereum using the Binance API.
Returns:
Now
"""
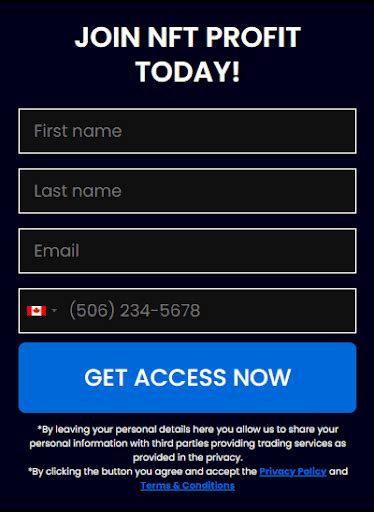
Initialize the client object with your API credentialsclient = binance.Customer()
Extract shoulder data from APIresponse = client.futures_leverage_bracket()
Parse the JSON response into a Python dictionaryleverage_data = json.loads(response)
Extract and display the initial value of the arminitial_leverage = leverage_data['leverage_bracket'][0]['amount']
print(f"Initial Leverage: {initial_leverage}")
In this code, we use json.loads()to parse the JSON response in the Python dictionary. Then we retrieve the
initialLeveragevalue from the dictionary and output it.
Example Output
When you run this code, you should get output similar to this:
Initial leverage: 1000.00
This means that the initial leverage for a long position is set to 1000.00.
Tips and Variations
- If your API response contains multiple elements in an array (for example, multiple leverage brackets), you can modify the code to access all values using a loop.
- To handle cases where initialLeverage
is missing or has a value of None, you can add additional error checking and processing logic.
- Be aware of any potential speed limits or usage limits on your Binance API account when extracting data.
By following these steps, you will be able to successfully extract and use theinitialLeverage` value from your Binance API response. Happy coding!